The WordPress Metadata is a powerful data structure that is being used across several parts of WordPress. That is why we have different tables that are used for storing the metadata. In this tutorial, I will teach you how to hook in the WordPress metadata and decide what to do with it.
If I love something about WordPress development, then it is the WordPress Plugin API. The amount of flexibility it gives you to hook in any part of WordPress and control the outcome it is just incredible.
Have you ever wondered about the possibility of hooking into WordPress metadata and change how the data is saved? Why not log a simple note when something important got changed?
The idea for this topic came from a discussion with Goran Jakovljevic, the developer behind the Swifty Bar (the fixed bar you see here). He wanted to disable a plugin to save some data from another plugin.
The WordPress Metadata
Before we dive into hooks and learn what filters and actions we can use, let’s just remind ourselves about the WordPress metadata. The WordPress core uses a few meta tables:
- Posts – postmeta, type: post
- Users – usermeta, type: user
- Comments – commentmeta, type: comment
- Terms – termmeta, type: term
All the meta tables follow the same table structure:
- meta_id
- {object}_id – different for each type
- meta_key
- meta_value
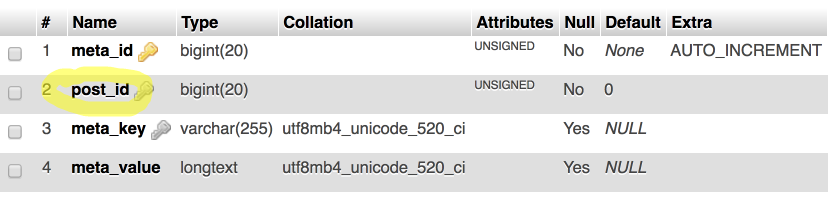
Object ID column is the post_id in postmeta table
Metadata CRUD Functions
The CRUD (Create, Read, Update, Delete) functions that work with the metadata table are:
For every WordPress metadata table, the core provides you with wrapper functions such as add_post_meta for postmeta table.
Custom Metadata
WordPress does provide you a way to register your own meta tables. If you have a plugin that could use a metadata table, you can register your own and also create wrapper functions to handle the manipulation of your metadata.
When creating your own custom metadata, you must:
- follow the same structure of the other WordPress Metadata tables,
- register the new table to the global
$wpdb
- create wrapper functions
I will not go into that now because that is an entire article on its own. Pippin Williamson has a great article on extending the metadata API.
Adding WordPress Metadata
When using functions such as add_post_meta
or add_user_meta
, we are actually calling the function add_metadata
. This function has several hooks inside that can be used to create additional events. Let’s see all those hooks:
The first hook is a filter. This filter will stop the data from being saved if we return something other than null
. Here is an example of hooking a user meta table & checking if the data should be saved or not.
The other two hooks are actions. This actions will trigger before the data is saved and also after. Let’s now see how we can combine those 2 hooks to implement a solution where someone tries to change their nickname to admin. We won’t allow it and we will change the nickname to something else:
In this events, we are first emailing our administrator of the user who wants to change his/her nickname to admin. After it has been saved, we are changing the nickname to something else.
Updating WordPress Metadata
When updating a metadata record, we can use similar hooks as in the previous examples:
As you can see, for post meta, there are also 2 custom actions added in the WordPress core already. Each action here provides us also with the $meta_id
which was not the case in all actions when adding occurs.
If you are hooking to this functions, be sure to not use the same function inside the hook since then you could end up with an infinite loop:
WordPress does have a nice check on the current and previous value we want to update, so if they are the same, the function will return false. This will make sure we don’t end up in an infinite loop. But, what if we create random values? We could end up updating our user meta indefinitely.
Getting WordPress Metadata
The function get_metadata
is pretty simple. It does not contain any actions and it uses only 1 filter. This filter is almost the same as the filters in the previous examples. The difference is that if the $check
is not null, that value will be returned.
This filter can then be used if we need to intercept the value of the $meta_key
and return something different. This can be pretty powerful in some situations. Here is just a simple example to show you how you can utilize this filter to return something else.
Deleting WordPress Metadata
This is the last part of the CRUD functions. To delete a WordPress metadata, we are utilizing the function delete_metadata
.
This function has the same hooks as the previous ones for adding and updating:
The filter provides us with the variable $delete_all
that indicates if WordPress is going to delete all the metadata with the provided $meta_key
or just to delete the metadata with the provided $object_id
. Here is an example where we block the deletion of all nicknames at once:
Other actions do pass the parameter $meta_ids
. This parameter is not a single metadata ID, but an array of all the metadata IDs that are going to be deleted. If you need to do something about them before or after they are deleted, you need to a for
or foreach
loop on them and perform the function.
Conclusion
WordPress Plugin API is really extraordinary. With this tutorial we have learned a lot and I have showed you how you hook into the WordPress metadata. Be aware that there are other functions there that you can also check and use when working with WordPress metadata. The functions can be viewed in the file wp-includes/meta.php
.
If you have ever hooked into an update function or any other that had to do with the WordPress metadata feel free to share it with us. I am eager to see many use of this hooks:)
If you are interested in becoming a WordPress developer, you can check my 7-day free email course.
Become a Sponsor
Best article I’ve read on the topic of WordPress meta data. Very well written and thorough with good examples. Thanks!
Thank you, Damien for your comment! Really glad you like it!
Thanks, It was very useful for me